Kift
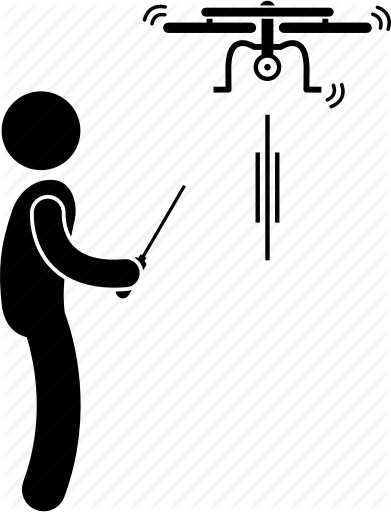
Thrift(v0.12.0) plugin for Kerbal Space Program(v1.7.3+).
Doc portal: https://vaporz.github.io/Kift/index.html
I tried to use GRPC at first, but it appears that GRPC requires .NET Framework 4.5+, and unfortunately, KSP is built on .NET Framework 3.5, which is quite old.
What is Kift?
Kift is inpired by kRPC -- a great Mod from @djungelorm.
Kift is a bridge between your code and the game, you can run your own codes to control the game from outside of the game.
Architecture
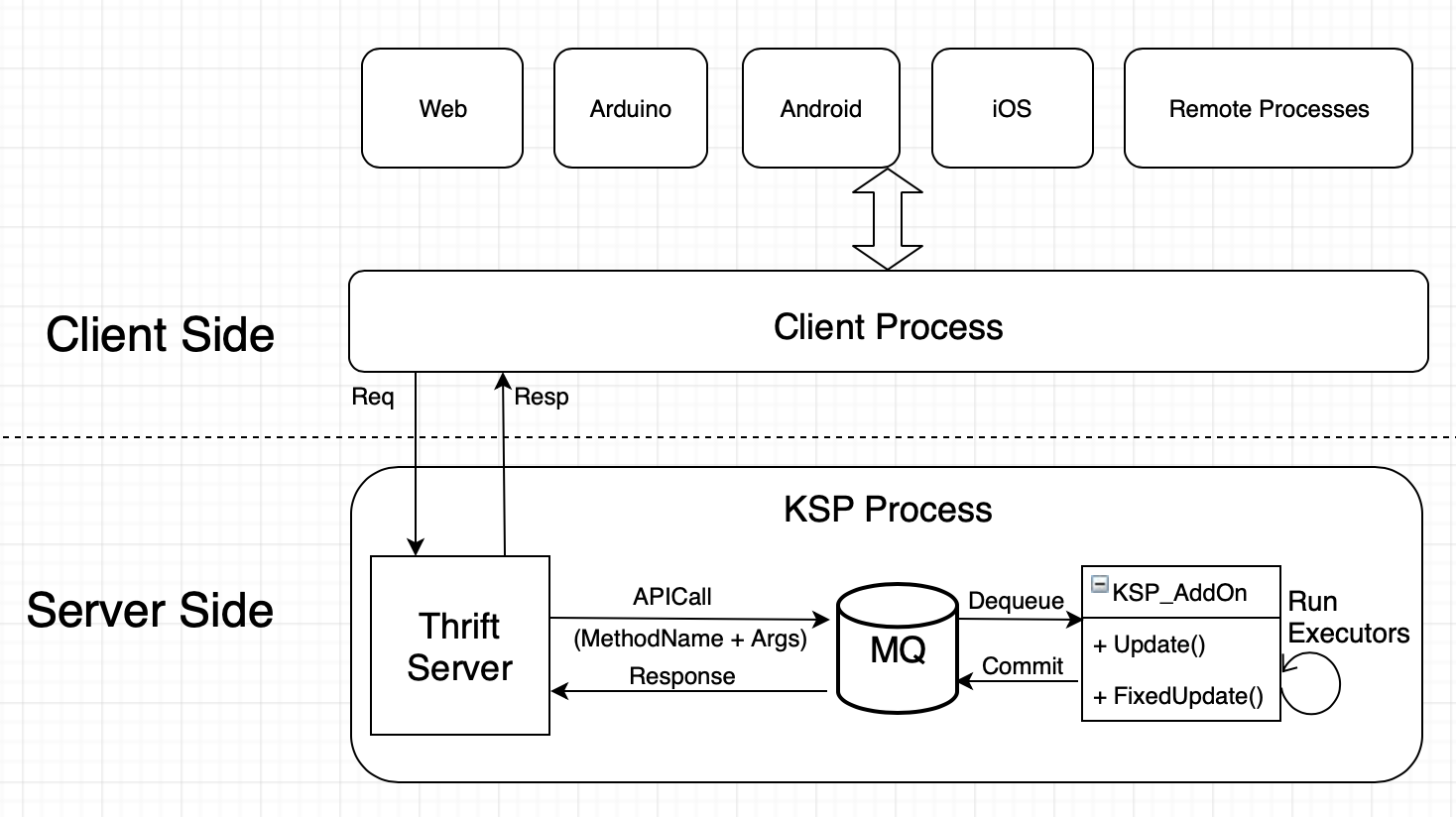
Kift starts a Thrift server inside of KSP process. The server receives requests, then send them to a Message Queue. A KSP addon will dequeue requests from the queue, and run the corresponding Executor. The server will wait until the response to a request is returned.
Supported languages
Theoritically, any programming language supported by Thrift v0.12.0 is supported by Kift.
Languages supported by Thrift v0.12.0:
C, C++, Go, Java, Python, PHP, Ruby, Erlang, Perl, Haskell, C#, Cocoa, JavaScript, Node.js, Smalltalk, OCaml and Delphi, etc.
Getting Started
1, Install Kift
Download Kift.zip, copy the "Kift" folder in zip file into "Kerbal Space Programe/GameData" directory.
Now the server side is ready. When your game is loaded and the main menu shows up, the Thift server is started and listening for requests.
2, Connect to Thrift server, and call Kift APIs
To interact with the Thrift server within KSP process, you have to write your own client side codes.
Following lines of code show how to call a Kift API to get current vessel's info, in C#:
// The game is started, and MUST be in Flight scene.
// Import "Kift.ThriftGen.dll"(built from "Kift/thrift-gen/gen-csharp"),
// or generate stub codes by yourself, then build and import your DLL file.
using Kift;
// Import "Thrift.dll": https://cwiki.apache.org/confluence/display/thrift/ThriftUsageCSharp
using Thrift.Protocol;
using Thrift.Transport;
...
// The port is configable in "Kerbal Space Program/GameData/Kift/server.cfg"
TTransport transport = new TFramedTransport(new TSocket("localhost", 9090));
TProtocol protocol = new TCompactProtocol(transport);
KiftService.Client client = new KiftService.Client(protocol);
transport.Open(); // Connect to server
Vessel v = client.currentVessel(); // Call API "currentVessel"
Console.WriteLine(v.Name);
...
transport.Close(); // finally close the connection
Kift APIs
Kift provides two sets of APIs: "InfoSync APIs" and "Command APIs".
"InfoSync APIs" pull infos from the game. "Command APIs" send commands to control vessels/parts/game.
Command APIs are devided into three categories according to the target.
- InfoSync APIs
- Real time snapshot
Read data at real time(approximately), useful when you want to monitor any data, such as height, speed, etc. - GetInfo APIs
Get data from current game, such as current vessel, the part list of a vessel, etc.
- Real time snapshot
- Command APIs
- Vessel Control APIs
APIs to control a vessel, such as pitch/yaw/roll, enable/disable RCS, deploy/restract landing legs, etc. - Part Control APIs
APIs to control a part, such as deploy/restract a solar panel, open/close a cargo bay, etc. - Game Control APIs
APIs to control the game, such as time warp, change camera mode, set/remove maneuver nodes etc.
- Vessel Control APIs
See more about Kift APIs at the doc portal: https://vaporz.github.io/Kift/api/index.html
Generate Thrift stub code for other languages
There're ready-to-use stub codes under "Kift/thrift-gen", including:
C, C++, C#, Go, Java, JavaScript, Node.js, Lua, PHP, Ruby and Rust.
Just import the stub code to your project, then you can create a client to connect to server, and eventually call Kift APIs.
If the language you are using is not in the list, you can generate thrift stub codes by yourself.
Thrift is a software framework, for scalable cross-language services development. With the help of Thrift, you can write client code in any programming language, as long as it's supported by Thrift.
Kift APIs are defined in IDL file "service.thrift" in folder "Kift/thrift-gen".
Download a copy of Thrift v0.12.0, then generate stub codes with following command:
thrift --gen <language> <Thrift filename>
For example, if you're using C#, then generate C# stub codes with
$thrift -r --gen csharp service.thrift
if you're using Golang, then run
$thrift -r --gen go service.thrift
Some notes
- To Kift developers: Copy "Kerbal Space Program" folder into "Kift" folder.
- Any feature which can be implemented with exsiting APIs is not likely to be added to Kift APIs, for example, autopilot.
- Advanced features are supposed to be implemented at client side, the code are under directory "Kift/client/[Language]".